The meaning of operators are already defined and fixed for basic
types like: int, float, double etc in C++ language. For example: If you
want to add two integers then, + operator is used. But, for user-defined
types(like: objects), you can define the meaning of operator, i.e, you
can redefine the way that operator works. For example: If there are two
objects of a class that contain string as its data member, you can use +
operator to concatenate two strings. Suppose, instead of strings if
that class contains integer data member, then you can use + operator to
add integers. This feature in C++ programming that allows programmer to
redefine the meaning of operator when they operate on class objects is
known as operator overloading.
The return type comes first which is followed by keyword operator,
followed by operator sign,i.e., the operator you want to overload like:
+, <, ++ etc. and finally the arguments is passed. Then, inside the
body of you want perform the task you want when this operator function
is called.
This operator function is called when, the operator(sign) operates on the object of that class class_name.
In this program, a operator function
Again, the above example is increase count by 1 is not complete. This program is incomplete in sense that, you cannot use code like:
Here, are the examples of operator overloading on different types of operators in C++ language in best possible ways:
This is not all in Operator Overloading... I will upload some more stuff on it very soon..
Why Operator overloading is used in C++ programming?
You can write any C++ program without the knowledge of operator overloading. But, operator operating are profoundly used by programmer to make a program clearer. For example: you can replace the code like: calculation = add(mult(a,b),div(a,b));
with calculation = a*b+a/b;
which is more readable and easy to understand.How to overload operators in C++ programming?
To overload a operator, a operator function is defined inside a class as: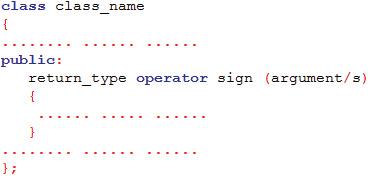
This operator function is called when, the operator(sign) operates on the object of that class class_name.
Example of operator overloading in C++ Programming
/* Simple example to demonstrate the working of operator overloading*/
#include <iostream>
using namespace std;
class temp
{
private:
int count;
public:
temp():count(5){ }
void operator ++() {
count=count+1;
}
void Display() { cout<<"Count: "<<count; }
};
int main()
{
temp t;
++t; /* operator function void operator ++() is called */
t.Display();
return 0;
}
Output
Count: 6
ExplanationIn this program, a operator function
void operator ++ ()
is defined(inside class temp), which is invoked when ++ operator operates on the object of type temp. This function will increase the value of count by 1.Things to remember while using Operator overloading in C++ language
- Operator overloading cannot be used to change the way operator works on built-in types. Operator overloading only allows to redefine the meaning of operator for user-defined types.
- There are two operators assignment operator(=) and address
operator(&) which does not need to be overloaded. Because these two
operators are already overloaded in C++ library. For example: If obj1 and obj2 are two objects of same class then, you can use code
obj1=obj2;
without overloading = operator. This code will copy the contents object of obj2 to obj1. Similarly, you can use address operator directly without overloading which will return the address of object in memory. - Operator overloading cannot change the precedence of operators and associativity of operators. But, if you want to change the order of evaluation, parenthesis should be used.
- Not all operators in C++ language can be overloaded. The operators that cannot be overloaded in C++ are ::(scope resolution), .(member selection), .*(member selection through pointer to function) and ?:(ternary operator).
Following best practice while using operator overloading
Operator overloading allows programmer to define operator the way they want but, there is a pitfall if operator overloading is not used properly. In above example, you have seen ++ operator operates on object to increase the value of count by 1. But, the value is increased by 1 because, we have used the code:void operator ++() {
count=count+1;
}
If the code below was used instead, then the value of count will be decreased by 100 if ++ operates on object.void operator ++() {
count=count-100;
}
But, it does not make any sense to decrease count by 100 when
++ operator is used. Instead of making code readable this makes code
obscure and confusing. And, it is the job of the programmer to use
operator overloading properly and in consistent manner.Again, the above example is increase count by 1 is not complete. This program is incomplete in sense that, you cannot use code like:
t1=++t
It is because the return type of operator function
is void. It is generally better to make operator work in similar way it
works with basic types if possible.Here, are the examples of operator overloading on different types of operators in C++ language in best possible ways:
This is not all in Operator Overloading... I will upload some more stuff on it very soon..